Thursday, January 26, 2006
Due: Wednesday, February 1, 2006 - 10:00PM
Preliminaries
Today you will work on setting up a simple GUI (graphical user interface) for BankAccount objects -- a mini-ATM program, if you will. By the end of the lab, your program should look like this:
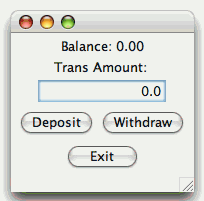
Lab Exercises
1.
In your Eclipse workspace, start a new project called "lab03". Copy the following code file into your project: BankAccount.java
2.
Set up the component and frame classes for the GUI application. Create a new class called "MiniATM" with a main method that sets up a JFrame with the title "MiniATM" and makes the frame visible. Create another class called "MiniATMComponent" that extends JComponent. The main method should add a MiniATMComponent object to the frame before it displays it. (Hint: Instead of writing all this from scratch, copy the code in some other pair of "...Viewer"/"...Component" classes -- like what we worked on in class yesterday.
3.
Add the following fields to the MiniATMComponent class:
// constant for the size of the input field private int AMT_FIELD_WIDTH = 10; // The bank account object itself private BankAccount acct; // user interface components private JLabel balanceLabel; private JTextField amtField; private JButton depositButton; private JButton withdrawButton; private JButton exitButton;
Add a constructor to the MiniATMComponent class that constructs and adds the user interface components to a JPanel which is then added to the component. (See the ButtonTester example we did in class.) Look up the documentation for the various component classes in the Java API reference to see how to call their constructors (or look at the examples in the textbook on page 448-449).
The constructor should also initialize the bank account object with an initial balance of 0.00.
4.
Set up event listeners for the three buttons. You can do this by defining inner class(es) that implement the ActionListener interface. You can either define a single inner class with an actionPerformed method that checks to see which button was pressed, or you can define three different classes to handle the different events.
The listener for the Exit button should simply make a call to
System.exit(0);
to exit the program.The event handling code for the Deposit button should parse the value in the amtField text field (using the getText() and Double.parseDouble methods) and then deposit the money into the bank account. The text of the balanceLabel text label should be updated to reflect the new balance. You can use this statement to do so:
balanceLabel.setText( String.format("Balance: %.2f", acct.getBalance()));
. After depositing into the account, the text of the amtField should also be set back to "0.00".The event handling code for the Withdraw button should work similarly as the deposit button, but call the withdraw method on the BankAccount object.
When you have your application done and running, show it to me.
Prepare for class tomorrow
In preparation for our class tomorrow, download the three Game-of-Life source code files from the links on the schedule page and bring them into your Eclipse "lecture3" project. Look over the code and run the program to see what it does. This code is based on Project 8.2, page 320, in the textbook. In class we will work on adding event listeners so that you can use the mouse to turn on/off the state of cells.
Homework Exercises
Again, read the following article carefully and take it to heart: How NOT to go about a programming assignment. If I only start hearing from you about problems you're having with the assignment on Wednesday afternoon, I'll know you didn't read all the way to the bottom of this article.
[60 points] Complete Programming Project 12.2 on page 464. We will learn how to handle mouse clicks in a component in the next class or two. You can get started before then, by designing a class(es) that will add points to their set of data and plot the regression line. Although it's true, as the book mentions, that you don't need to keep the entire list of points to compute the regression statistics, it would probably still be beneficial to keep an ArrayList of points so that they can be plotted on the window along with the regression line itself.
Note: All source code that you produce must conform to the following style guide, adapted from the textbook's Appendix A: Java Programming Style Guide
Your completed source code files should be turned into the proper submit folder (submit03) by the deadline.